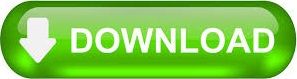
Here's an example of the syntax for this function: Python allows you to accomplish that task by using the "merge_pdfs()" function.
PDFWRITER MODULE PYTHON PDF
For instance, you can merge a standard cover page with various PDF reports. There are various circumstances when you may want to merge multiple PDFs into a single PDF. # rotate_pages.py from PyPDF2 import PdfFileReader, PdfFileWriter def rotate_pages(pdf_path): pdf_writer = PdfFileWriter() pdf_reader = PdfFileReader(pdf_path) # Rotate page 90 degrees to the right page_1 = pdf_reader.getPage(0).rotateClockwise(90) pdf_writer.addPage(page_1) # Rotate page 90 degrees to the left page_2 = pdf_reader.getPage(1).rotateCounterClockwise(90) pdf_writer.addPage(page_2) # Add a page in normal orientation pdf_writer.addPage(pdf_reader.getPage(2)) with open('rotate_pages.pdf', 'wb') as fh: pdf_writer.write(fh) if _name_ = '_main_': path = 'Jupyter_Notebook_An_Introduction.pdf' rotate_pages(path) 3.
PDFWRITER MODULE PYTHON HOW TO
Here's an example of the syntax showing you how to rotate pages correctly using this library:
PDFWRITER MODULE PYTHON INSTALL
To rotate PDF pages, install the PyPDF2 library by inputting the command "pip install pypdf2". Sometimes, the pages may be upside down, which can occur when someone scans a document to a PDF or their email. Pages in a PDF may either be in landscape or portrait mode. Related: How To Write on a PDF Using 3 Different Methods 2. Here's an example syntax for adding text to your PDF file:įrom import LETTER from import inch from import Canvas from import red # creating the pdf file canvas = Canvas("text_file.pdf", pagesize=LETTER) # setting up the font and the font size tFont("Courier", 16) # setting up the color of the font as red tFillColor(red) # writing this text on the PDF file canvas.drawString(2 * inch, 8 * inch, "This is a newly created Python PDF.") canvas.save() Here's an example showing the syntax for extracting text from a PDF in Python using this library:įrom io import StringIO from pdfminer.pdfinterp import PDFPageInterpreter, PDFResourceManager from pdfminer.pdfpage import PDFPage from nverter import TextConverter from pdfminer.layout import LAParams # PDFMiner Analyzers rsrcmgr = PDFResourceManager() sio = StringIO() codec = "utf-8" laparams = LAParams() device = TextConverter(rsrcmgr, sio, codec=codec, laparams=laparams) interpreter = PDFPageInterpreter(rsrcmgr, device) # path to our input file pdf_file = "sample.pdf" # Extract text pdfFile = open(pdf_file, "rb") for page in PDFPage.get_pages(pdfFile): interpreter.process_page(page) fp.close() # Return text from StringIO text = sio.getvalue() print(text) # Freeing Up device.close() sio.close() Once the library installs, you can add and extract text from a PDF. To extract text from Python, install PDFMiner by running the command "pip install pdfminer.six". Here are nine unique ways you can work with PDF files in Python: 1. In this article, we discuss nine ways you can use PDFs in Python to help you enhance your programming skills for your career. Learning these modification techniques can help you personalize your files to meet your specific needs and requirements. When programming with Python, you can use different libraries and functions to customize PDF files such as watermarking and highlighting sections of a document. RestPdfRStreams.forEach(pdfRStream => pdfWriter.Python is an intuitive programming tool that offers a diverse range of features to help developers write and edit code. createWriterToModify shouldn't require any PDF streamĬonst = pdfBlobs.map(pdfBlob => new PDFRStreamForBuffer(pdfBlob)) Ĭonst pdfWriter = createWriterToModify(firstPdfRStream, new PDFStreamForResponse(outStream)) Hummus is useful, but with poor interfaces - E.g. If (pdfBlobs.length = 1) return pdfBlobs This optimization is not necessary, but it avoids the churn down below
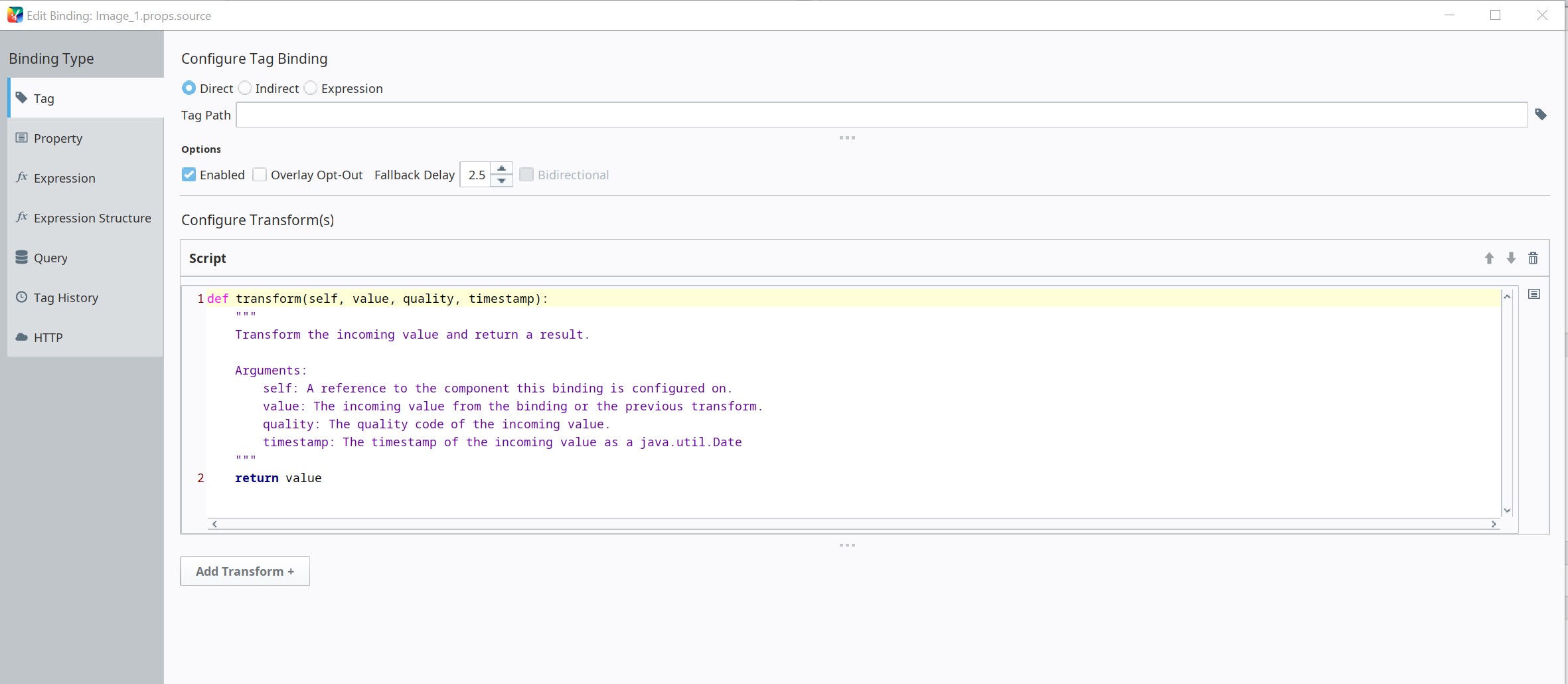
If (pdfBlobs.length = 0) throw new Error('mergePdfs called with empty list of PDF blobs')

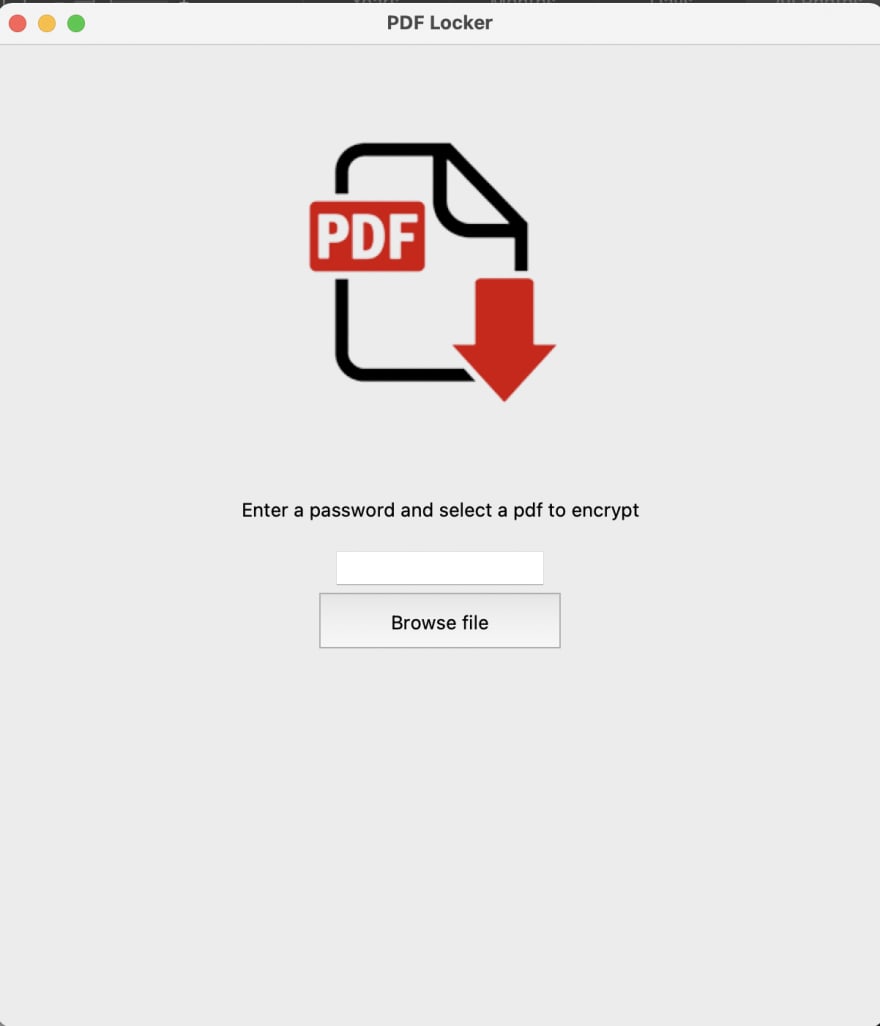
Merge the pages of the pdfBlobs (Javascript buffers) into a single PDF blob
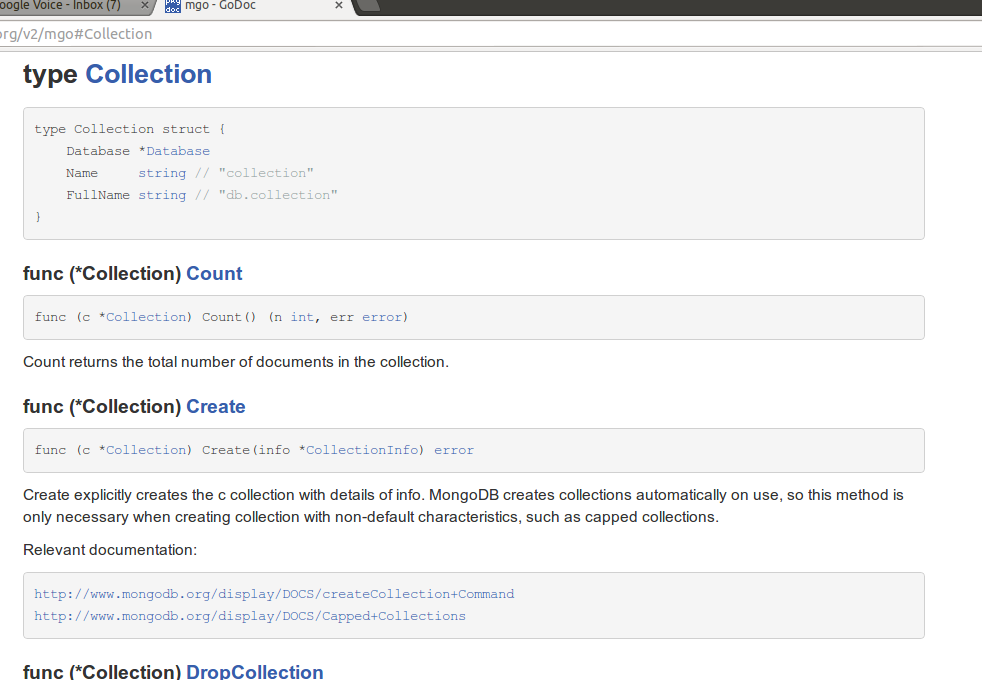
HummusJS supports combining PDFs using its appendPDFPagesFromPDF methodĮxample using streams to work with buffers: const hummus = require('hummus') Ĭonst memoryStreams = require('memory-streams')
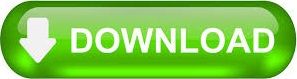